The muon stopping sites are determined using a well defined protocol:
1 - Generate a number of initial muon interstitial sites.
2 - Execute the supercell (SC) convergence sub-workflow (see IsolatedImpurityWorkChain
of the aiida
4 - Inspect and ensure that at least 60% of the simulations of step III are completed successfully; if not, the workflow stops due to structural convergence failure.
5 - Collect the relaxed structures and their total energies, and cluster distinct stable structures on the basis of symmetry and total energy differences (see ``Unique sites selection’’ section).
Notably, thanks to the fault tolerant and fault resilient algorithms of the aiida-quantumespresso
PwBaseWorkChain
, the workflow in step III can handle a range of typical errors, such as unconverged SCF calculations or hitting of walltime limits, ensuring that in most cases the calculations finish successfully. In step V, for magnetic compounds, it can happen that crystallographically equivalent replica of a candidate muon site may be magnetically inequivalent. When this happens, step III is reactivated, so that relaxed structures of missing magnetically inequivalent sites are obtained and added to the list. Calculations for the charged supercell for the the Mu+ state (default) and neutral for the \Mudot~state (optional) are run independently and controlled in the workflow by the Boolean input parameter charged_supercell.
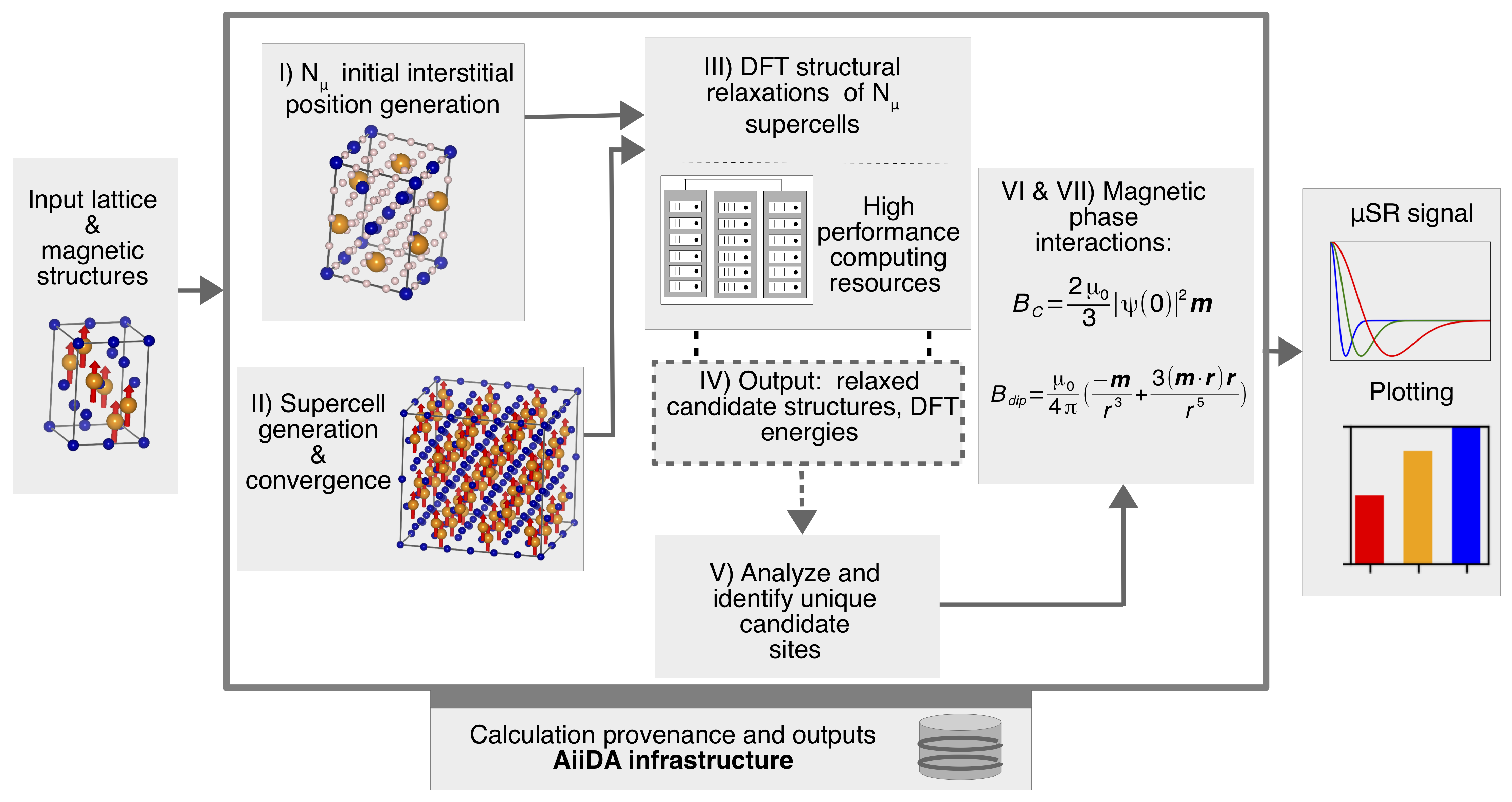
How to prepare and run a simple FindMuonWorkChain
¶
# Loading the aiida profile.
from aiida import load_profile, orm
load_profile()
# Loading other additional aiida modules.
from aiida.engine import submit, run_get_node
from aiida.plugins import DataFactory
# Loading the pymatgen Structure module; not mandatory.
from pymatgen.core import Structure
# Loading the WorkChain.
from aiida_muon.workflows.find_muon import FindMuonWorkChain
Setting the data¶
Here below we parse the structure, the magnetic moments, and we set some other relevant inputs for the workflow:
- sc_matrix=[[[1, 0, 0], [0, 1, 0], [0, 0, 1]]]
- mu_spacing=2.0
- kpoints_distance=0.801
- charge_supercell = True
# StructureData and magmom.
smag1 = Structure.from_file("../examples/data/Fe_bcc.mcif", primitive=False)
aiida_structure = orm.StructureData(pymatgen=smag1)
magmoms = smag1.site_properties["magmom"]
magmom = [list(magmom) for magmom in magmoms]
# Supercell Matrix and other relevant inputs.
sc_matrix = [[1, 0, 0], [0, 1, 0], [0, 0, 1]]
mu_spacing =2.0
kpoints_distance = 0.801
charge_supercell = True
# Codes.
codename = "pw-7.2@localhost" # edit
code = orm.load_code(codename)
pp_codename = "pp-7.2@localhost" # edit
pp_code = orm.load_code(pp_codename)
# Resources dictionary with a minimal computational settings.
resources = {
"num_machines": 1,
"num_mpiprocs_per_machine": 8,
}
(1) Getting the builder from get_builder_from_protocol() function.¶
builder = FindMuonWorkChain.get_builder_from_protocol(
pw_code=code,
pp_code = pp_code,
structure = aiida_structure,
magmom = magmom,
#sc_matrix = sc_matrix,
mu_spacing = mu_spacing,
kpoints_distance = kpoints_distance,
charge_supercell = charge_supercell,
pseudo_family = "SSSP/1.3/PBE/efficiency"
)
builder.pwscf.pw.metadata.options.resources = resources
builder.pwscf.pw.metadata.options.prepend_text = "export OMP_NUM_THREADS=1"
builder.relax.base.pw.metadata.options.resources = resources
builder.relax.base.pw.metadata.options.prepend_text = "export OMP_NUM_THREADS=1"
builder.impuritysupercellconv_metadata = {"options":{
'resources':resources,
'prepend_text':"export OMP_NUM_THREADS=1",
},}
builder.pp_metadata = {"options":{
'resources':resources,
'prepend_text':"export OMP_NUM_THREADS=1",
},}
submit the workchain, just run:
submission = run_get_node(builder)
How to parse results of a FindMuonWorkChain
¶
As outputs of the WorkChain, results are collected in several nodes:
- all_index_uuid:
- all_sites:
- unique_sites:
- unique_sites_hyperfine:
- unique_sites_dipolar ; returns only when magnetic.